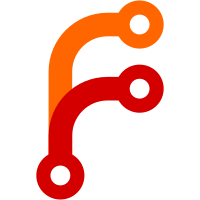
If the user tries to run a program that doesn't exist from Bash, the program name is looked up in a database that maps to Nix package names. If it is found, we print out a message like: $ pdflatex The program ‘pdflatex’ is currently not installed. It is provided by several packages. You can install it by typing one of the following: nix-env -i tetex nix-env -i texlive-core If the environment variable $NIX_AUTO_INSTALL is set, the command is installed and executed automatically: $ hello --version The program ‘hello’ is currently not installed. It is provided by the package ‘hello’, which I will now install for you. installing `hello-2.8' hello (GNU hello) 2.8 Copyright (C) 2011 Free Software Foundation, Inc. ... To use this, you must currently manually put the SQLite programs database in /var/lib/nixos/programs.sqlite. In the future, this file should be provided as part of the NixOS channel so it gets updated automatically. To get a test version: $ curl http://nixos.org/~eelco/programs.sqlite.xz | xz -d > /var/lib/nixos/programs.sqlite
49 lines
1.3 KiB
Perl
49 lines
1.3 KiB
Perl
#! @perl@/bin/perl -w @perlFlags@
|
||
|
||
use strict;
|
||
use DBI;
|
||
use DBD::SQLite;
|
||
use Config;
|
||
|
||
my $program = $ARGV[0];
|
||
|
||
my $dbPath = "/var/lib/nixos/programs.sqlite";
|
||
|
||
my $dbh = DBI->connect("dbi:SQLite:dbname=$dbPath", "", "")
|
||
or die "cannot open database `$dbPath'";
|
||
$dbh->{RaiseError} = 0;
|
||
$dbh->{PrintError} = 0;
|
||
|
||
my $system = $ENV{"NIX_SYSTEM"} // $Config{myarchname};
|
||
|
||
my $res = $dbh->selectall_arrayref(
|
||
"select package from Programs where system = ? and name = ?",
|
||
{ Slice => {} }, $system, $program);
|
||
|
||
if (!defined $res || scalar @$res == 0) {
|
||
print STDERR "$program: command not found\n";
|
||
} elsif (scalar @$res == 1) {
|
||
my $package = @$res[0]->{package};
|
||
if ($ENV{"NIX_AUTO_INSTALL"} // "") {
|
||
print STDERR <<EOF;
|
||
The program ‘$program’ is currently not installed. It is provided by
|
||
the package ‘$package’, which I will now install for you.
|
||
EOF
|
||
;
|
||
exit 126 if system("nix-env", "-i", $package) == 0;
|
||
} else {
|
||
print STDERR <<EOF;
|
||
The program ‘$program’ is currently not installed. You can install it by typing:
|
||
nix-env -i $package
|
||
EOF
|
||
}
|
||
} else {
|
||
print STDERR <<EOF;
|
||
The program ‘$program’ is currently not installed. It is provided by
|
||
several packages. You can install it by typing one of the following:
|
||
EOF
|
||
print STDERR " nix-env -i $_->{package}\n" foreach @$res;
|
||
}
|
||
|
||
exit 127;
|