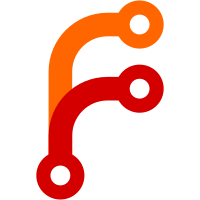
boots into stage 1 (kernel+initrd) succesfully. `system-configuration.nix' contains the definition of the configuration to be installed. The attribute systemConfiguration is installed into the profile /nix/var/nix/profiles/system. Then the program /nix/var/nix/profiles/system/bin/switch-to-configuration is called to finalise the installation. This program (generated by system-configuration.sh) installs Grub on the drive with a menu that contains the entry for the desired kernel and initrd. In principle this allows us to do rollbacks to previous system configurations by doing `nix-env --rollback' and then calling switch-to-configuration to update Grub. Ideally this should be done in a single command (and we should consider the obvious risk of garbage collecting the current kernel etc. to which the current Grub menu points...). Maybe the responsibility for generating the Grub menu should be placed somewhere else. For instance, we could generate a Grub menu automatically out of all the generations in the `system' profile. svn path=/nixu/trunk/; revision=7009
135 lines
3.5 KiB
Bash
135 lines
3.5 KiB
Bash
#! @shell@
|
|
|
|
# Syntax: installer.sh <DEVICE> <NIX-EXPR>
|
|
# (e.g., installer.sh /dev/hda1 ./my-machine.nix)
|
|
|
|
# - mount target device
|
|
# - make Nix store etc.
|
|
# - copy closure of rescue env to target device
|
|
# - register validity
|
|
# - start the "target" installer in a chroot to the target device
|
|
# * do a nix-pull
|
|
# * nix-env -p system-profile -i <nix-expr for the configuration>
|
|
# * run hook scripts provided by packages in the configuration?
|
|
# - install/update grub
|
|
|
|
set -e
|
|
|
|
targetDevice="$1"
|
|
nixExpr="$2"
|
|
|
|
if test -z "$targetDevice" -o -z "$nixExpr"; then
|
|
echo "syntax: installer.sh <targetDevice> <nixExpr>"
|
|
exit 1
|
|
fi
|
|
|
|
nixExpr=$(readlink -f "$nixExpr")
|
|
|
|
|
|
# Make sure that the target device isn't mounted.
|
|
umount "$targetDevice" 2> /dev/null || true
|
|
|
|
|
|
# Check it.
|
|
fsck -n "$targetDevice"
|
|
|
|
|
|
# Mount the target device.
|
|
mountPoint=/tmp/inst-mnt
|
|
mkdir -p $mountPoint
|
|
mount "$targetDevice" $mountPoint
|
|
|
|
mkdir -p $mountPoint/dev $mountPoint/proc $mountPoint/sys $mountPoint/mnt
|
|
mount --rbind / $mountPoint/mnt
|
|
mount --bind /dev $mountPoint/dev
|
|
mount --bind /proc $mountPoint/proc
|
|
mount --bind /sys $mountPoint/sys
|
|
|
|
cleanup() {
|
|
for i in $(grep -F "$mountPoint" /proc/mounts \
|
|
| perl -e 'while (<>) { /^\S+\s+(\S+)\s+/; print "$1\n"; }' \
|
|
| sort -r);
|
|
do
|
|
umount $i
|
|
done
|
|
}
|
|
|
|
trap "cleanup" EXIT
|
|
|
|
mkdir -p $mountPoint/tmp
|
|
mkdir -p $mountPoint/var
|
|
|
|
|
|
# Create the necessary Nix directories on the target device, if they
|
|
# don't already exist.
|
|
mkdir -p \
|
|
$mountPoint/nix/store \
|
|
$mountPoint/nix/var/nix/gcroots \
|
|
$mountPoint/nix/var/nix/temproots \
|
|
$mountPoint/nix/var/nix/manifests \
|
|
$mountPoint/nix/var/nix/userpool \
|
|
$mountPoint/nix/var/nix/profiles \
|
|
$mountPoint/nix/var/nix/db \
|
|
$mountPoint/nix/var/log/nix/drvs
|
|
|
|
|
|
# Copy Nix to the Nix store on the target device.
|
|
echo "copying Nix to $targetDevice...."
|
|
for i in $(cat @nixClosure@); do
|
|
echo " $i"
|
|
rsync -a $i $mountPoint/nix/store/
|
|
done
|
|
|
|
|
|
# Register the paths in the Nix closure as valid. This is necessary
|
|
# to prevent them from being deleted the first time we install
|
|
# something. (I.e., Nix will see that, e.g., the glibc path is not
|
|
# valid, delete it to get it out of the way, but as a result nothing
|
|
# will work anymore.)
|
|
for i in $(cat @nixClosure@); do
|
|
echo $i
|
|
echo # deriver
|
|
echo 0 # nr of references
|
|
done \
|
|
| chroot $mountPoint @nix@/bin/nix-store --register-validity
|
|
|
|
|
|
# Create the required /bin/sh symlink; otherwise lots of things
|
|
# (notably the system() function) won't work.
|
|
mkdir -p $mountPoint/bin
|
|
ln -sf $(type -tp sh) $mountPoint/bin/sh
|
|
|
|
|
|
# Enable networking in the chroot.
|
|
mkdir -p $mountPoint/etc
|
|
cp /etc/resolv.conf $mountPoint/etc/
|
|
|
|
|
|
# Do a nix-pull to speed up building.
|
|
nixpkgsURL=http://nix.cs.uu.nl/dist/nix/nixpkgs-0.11pre6984
|
|
#chroot $mountPoint @nix@/bin/nix-pull $nixpkgsURL/MANIFEST
|
|
|
|
|
|
# Build the specified Nix expression in the target store and install
|
|
# it into the system configuration profile.
|
|
|
|
#rm -rf $mountPoint/scratch
|
|
#mkdir $mountPoint/scratch
|
|
#curl $nixpkgsURL/nixexprs.tar.bz2 | tar xj -C $mountPoint/scratch
|
|
#nixpkgsName=$(cd $mountPoint/scratch && ls)
|
|
|
|
chroot $mountPoint @nix@/bin/nix-env \
|
|
-p /nix/var/nix/profiles/system \
|
|
-f "/mnt/$nixExpr" -i system-configuration
|
|
|
|
|
|
# Grub needs a mtab.
|
|
echo "$targetDevice / somefs rw 0 0" > $mountPoint/etc/mtab
|
|
|
|
|
|
# Switch to the new system configuration. This will install Grub with
|
|
# a menu default pointing at the kernel/initrd/etc of the new
|
|
# configuration.
|
|
echo "finalising the installation..."
|
|
chroot $mountPoint /nix/var/nix/profiles/system/bin/switch-to-configuration
|